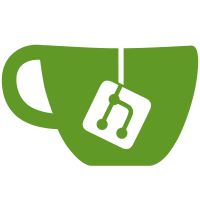
* update all READMEs with notices about the name change * update changelog for 0.24 * bump crate versions to 0.24 * update version notice information * update readmes to back reference trust-dns * rename all crates to hickory counterparts * replace all Trust-DNS references in code and comments with Hickory DNS * rename all Trust-DNS references to Hickory DNS in non-code * rename all trust-dns-resolver references to hickory-resolver * rename all trust-dns-client references to hickory-client * rename all trust-dns-proto references to hickory-proto * rename all trust-dns-server references to hickory-server * rename all trust-dns-compatibility references to hickory-compatability * rename all trust-dns-integration references to hickory-integration * rename all trust-dns-util references to hickory-util * Update MIT licenses to reference Hickory DNS * update all trust-dns references to hickory-dns * update all bluejekyll github references to hickorydns org * Update name in Changelog * make sure hickory-dns logs during tests * add changelogs for recent main additions * fix references to trust-dns and hickory in architecture * update a few trust-dns references in READMEs * fixup some dangling trust_dns references * replace fka with formerly in change log * replace all hickoydns org references to hickory-dns * replace all http links with https * update logos * update hickorydns to hickory-dns for all other org references * fix Notices of Trust-DNS to Hickory in each Readme
96 lines
3.2 KiB
Rust
96 lines
3.2 KiB
Rust
// Copyright 2015-2017 Benjamin Fry <benjaminfry@me.com>
|
|
//
|
|
// Licensed under the Apache License, Version 2.0, <LICENSE-APACHE or
|
|
// https://apache.org/licenses/LICENSE-2.0> or the MIT license <LICENSE-MIT or
|
|
// https://opensource.org/licenses/MIT>, at your option. This file may not be
|
|
// copied, modified, or distributed except according to those terms.
|
|
|
|
#![cfg(not(windows))]
|
|
#![cfg(feature = "dns-over-openssl")]
|
|
#![cfg(not(feature = "dns-over-rustls"))]
|
|
// TODO: enable this test for rustls as well using below config
|
|
// #![cfg(feature = "dns-over-tls")]
|
|
|
|
mod server_harness;
|
|
|
|
use std::env;
|
|
use std::fs::File;
|
|
use std::io::*;
|
|
use std::net::*;
|
|
|
|
use native_tls::Certificate;
|
|
use tokio::net::TcpStream as TokioTcpStream;
|
|
use tokio::runtime::Runtime;
|
|
|
|
use hickory_client::client::*;
|
|
use hickory_proto::native_tls::TlsClientStreamBuilder;
|
|
|
|
use hickory_proto::iocompat::AsyncIoTokioAsStd;
|
|
use server_harness::{named_test_harness, query_a};
|
|
|
|
#[test]
|
|
fn test_example_tls_toml_startup() {
|
|
test_startup("dns_over_tls.toml")
|
|
}
|
|
|
|
#[test]
|
|
fn test_example_tls_rustls_and_openssl_toml_startup() {
|
|
test_startup("dns_over_tls_rustls_and_openssl.toml")
|
|
}
|
|
|
|
fn test_startup(toml: &'static str) {
|
|
named_test_harness(toml, move |_, _, tls_port, _, _| {
|
|
let mut cert_der = vec![];
|
|
let server_path = env::var("TDNS_WORKSPACE_ROOT").unwrap_or_else(|_| "..".to_owned());
|
|
println!("using server src path: {}", server_path);
|
|
|
|
File::open(&format!(
|
|
"{}/tests/test-data/test_configs/sec/example.cert",
|
|
server_path
|
|
))
|
|
.expect("failed to open cert")
|
|
.read_to_end(&mut cert_der)
|
|
.expect("failed to read cert");
|
|
|
|
let mut io_loop = Runtime::new().unwrap();
|
|
let addr: SocketAddr = ("127.0.0.1", tls_port.expect("no tls_port"))
|
|
.to_socket_addrs()
|
|
.unwrap()
|
|
.next()
|
|
.unwrap();
|
|
let mut tls_conn_builder =
|
|
TlsClientStreamBuilder::<AsyncIoTokioAsStd<TokioTcpStream>>::new();
|
|
let cert = to_trust_anchor(&cert_der);
|
|
tls_conn_builder.add_ca(cert);
|
|
let (stream, sender) = tls_conn_builder.build(addr, "ns.example.com".to_string());
|
|
let client = AsyncClient::new(stream, sender, None);
|
|
let (mut client, bg) = io_loop.block_on(client).expect("client failed to connect");
|
|
hickory_proto::spawn_bg(&io_loop, bg);
|
|
|
|
query_a(&mut io_loop, &mut client);
|
|
|
|
let addr: SocketAddr = ("127.0.0.1", tls_port.expect("no tls_port"))
|
|
.to_socket_addrs()
|
|
.unwrap()
|
|
.next()
|
|
.unwrap();
|
|
let mut tls_conn_builder =
|
|
TlsClientStreamBuilder::<AsyncIoTokioAsStd<TokioTcpStream>>::new();
|
|
let cert = to_trust_anchor(&cert_der);
|
|
tls_conn_builder.add_ca(cert);
|
|
let (stream, sender) = tls_conn_builder.build(addr, "ns.example.com".to_string());
|
|
let client = AsyncClient::new(stream, sender, None);
|
|
let (mut client, bg) = io_loop.block_on(client).expect("client failed to connect");
|
|
hickory_proto::spawn_bg(&io_loop, bg);
|
|
|
|
// ipv6 should succeed
|
|
query_a(&mut io_loop, &mut client);
|
|
|
|
assert!(true);
|
|
})
|
|
}
|
|
|
|
fn to_trust_anchor(cert_der: &[u8]) -> Certificate {
|
|
Certificate::from_der(cert_der).unwrap()
|
|
}
|